The Label control represents a standard Windows label. It is generally used to display some informative text on the GUI, which is not changed during runtime.
Let's create a label by dragging a Label control from the Toolbox and dropping it on the form.
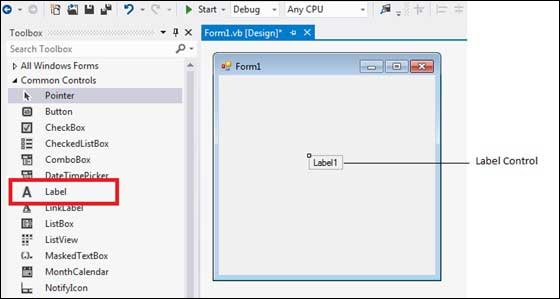
Properties of the Label Control
The following are some of the commonly used properties of the Label control:
S.N | Property | Description |
---|---|---|
1 | Autosize | Gets or sets a value specifying if the control should be automatically resized to display all its contents. |
2 | BorderStyle | Gets or sets the border style for the control. |
3 | FlatStyle | Gets or sets the flat style appearance of the Label control |
4 | Font | Gets or sets the font of the text displayed by the control. |
5 | FontHeight | Gets or sets the height of the font of the control. |
6 | ForeColor | Gets or sets the foreground color of the control. |
7 | PreferredHeight | Gets the preferred height of the control. |
8 | PreferredWidth | Gets the preferred width of the control. |
9 | TabStop | Gets or sets a value indicating whether the user can tab to the Label. This property is not used by this class. |
10 | Text | Gets or sets the text associated with this control. |
11 | TextAlign | Gets or sets the alignment of text in the label. |
Methods of the Label Control
The following are some of the commonly used methods of the Label control:
S.N | Method Name & Description |
---|---|
1 | GetPreferredSize Retrieves the size of a rectangular area into which a control can be fitted. |
2 | Refresh Forces the control to invalidate its client area and immediately redraw itself and any child controls. |
3 | Select Activates the control. |
4 | Show Displays the control to the user. |
5 | ToString Returns a String that contains the name of the control. |
Events of the Label Control
The following are some of the commonly used events of the Label control:
S.N | Event | Description |
---|---|---|
1 | AutoSizeChanged | Occurs when the value of the AutoSize property changes. |
2 | Click | Occurs when the control is clicked. |
3 | DoubleClick | Occurs when the control is double-clicked. |
4 | GotFocus | Occurs when the control receives focus. |
5 | Leave | Occurs when the input focus leaves the control. |
6 | LostFocus | Occurs when the control loses focus. |
7 | TabIndexChanged | Occurs when the TabIndex property value changes. |
8 | TabStopChanged | Occurs when the TabStop property changes. |
9 | TextChanged | Occurs when the Text property value changes. |
Consult Microsoft documentation for detailed list of properties, methods and events of the Label control.
Example
Following is an example which shows how we create two labels. Let us create the first label from the designer view tab and set its properties from the properties window. We will use the Click and the DoubleClick events of the label to move the first label and change its text and create the second label and add it to the form respectively.
Take the following steps:
- Drag and drop a Label control on the form.
- Set the Text property to provide the caption "This is a Label Control".
- Set the Font property from the properties window.
- Click the label to add the Click event in the code window and add the following codes.
Public Class Form1 Private Sub Form1_Load(sender As Object, e As EventArgs) _ Handles MyBase.Load ' Create two buttons to use as the accept and cancel buttons. ' Set window width and height Me.Height = 300 Me.Width = 560 ' Set the caption bar text of the form. Me.Text = "tutorialspont.com" ' Display a help button on the form. Me.HelpButton = True End Sub Private Sub Label1_Click(sender As Object, e As EventArgs) _ Handles Label1.Click Label1.Location = New Point(50, 50) Label1.Text = "You have just moved the label" End Sub Private Sub Label1_DoubleClick(sender As Object, e As EventArgs) Handles Label1.DoubleClick Dim Label2 As New Label Label2.Text = "New Label" Label2.Location = New Point(Label1.Left, Label1.Height + _ Label1.Top + 25) Me.Controls.Add(Label2) End Sub End Class
When the above code is executed and run using Start button available at the Microsoft Visual Studio tool bar, it will show following window:
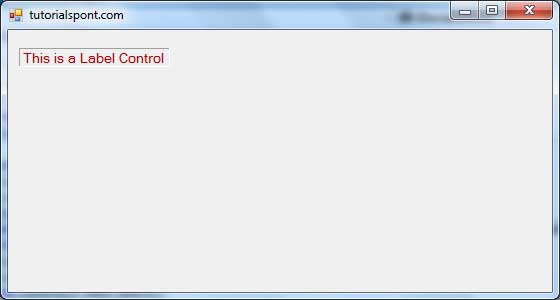
Clicking and double clicking the label would produce the following effect:
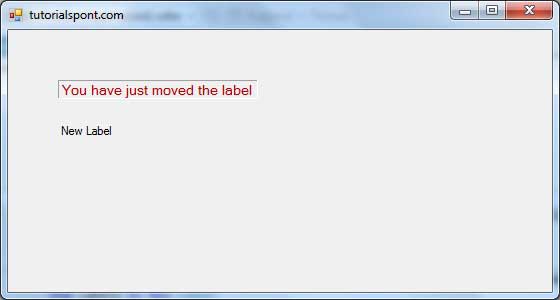
No comments:
Post a Comment